Using anonymous functions as callbacks
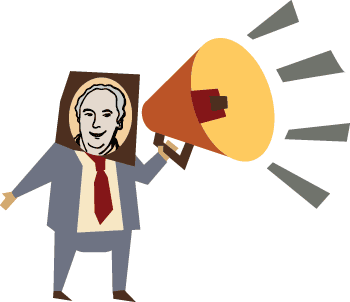
One common use of anonymous functions is to create simple inline callback functions. A callback function is a function that you create yourself, then pass to another function as an argument. Once it has access to your callback function, the receiving function can then call it whenever it needs to. This gives you an easy way to customise the behaviour of the receiving function.
Many built-in PHP functions accept callbacks, and you can also write your own callback-accepting functions. Let’s look at a couple of built-in functions that use callbacks, and see how to use them.
Using array_map(
) to run a callback function on each element in an array
PHP’s
array_map()
function accepts a callback function and an array as arguments. It then walks through the elements in the array. For each element, it calls your callback function with the element’s value, and your callback function needs to return the new value to use for the element. array_map()
then replaces the element’s value with your callback’s return value. Once it’s done, array_map()
returns the modified array.array_map()
works on a copy of the array you pass to it. The original array is untouched.
Here’s how you might use
array_map()
with a regular callback function:// Create a regular callback function... function nameToGreeting( $name ) { return "Hello " . ucfirst( $name ) . "!"; } // ...then map the callback function to elements in an array. $names = array( "fred", "mary", "sally" ); print_r( array_map( nameToGreeting, $names ) );
This code creates a regular function,
nameToGreeting()
, that takes a string, $name
, uppercases the first letter, prepends "Hello "
, and returns the result. Then, on line 8, the code passes this callback function to array_map()
, along with an array of names to work with, and displays the result:Array ( [0] => Hello Fred! [1] => Hello Mary! [2] => Hello Sally! )
While this code works, it’s a bit cumbersome to create a separate regular function just to act as a simple callback like this. Instead, we can create our callback as an inline anonymous function at the time we call
array_map()
, as follows:// A neater way: // Map an anonymous callback function to elements in an array. print_r ( array_map( function( $name ) { return "Hello " . ucfirst( $name ) . "!"; }, $names ) );
This approach saves a line of code, but more importantly, it avoids cluttering up the PHP file with a separate regular function that is only being used as a one-off callback.
No comments:
Post a Comment